DTO DSL
Contents
Purpose
The DTO DSL facilitates the creation and handling of data transfer objects (DTOs) in order to transfer data between processes. Communication between processes is usually done by calls to remote interfaces and services, where each call is a computationally expensive and time-consuming operation. The use of DTOs that aggregate data that would have been transferred separately reduces the number of calls that are necessary, thus speeding up the data-transfer operation. Furthermore, DTOs are decoupled from the JPA, thus eliminating burdensome dependencies.
Using the DTO DSL, it is easy to create DTOs and the mapper classes that link them to the respective persistence entities. Property-change support is automatically included, in order to have current data without direct dependencies on the persistence layer.
Overview
The main semantic elements of the DTO DSL are the following:
- package - The root element that contains all the other elements. A model can contain multiple packages.
- import declarations - Used to import other DTO models or the entity model files that are covered by the DTOs.
- datatype declarations - A way to define datatypes that can be used (only within the package - private scope).
- DTO - The model of a DTO that wraps an entity. It contains further elements such as properties and references. Appropriate mapper methods can be specified.
- property - A reference to an enum, a Java class or a “simple datatype" (as defined in the datatype declaration). Can be inherited from the wrapped entity and offers multiplicity.
- reference - A reference to another DTO. Can be inherited from the wrapped entity and offers multiplicity.
- operation - Similar to Java methods. The Xbase expression language can be used to write high-level code.
- comment - Can be added to all elements.
► Note: In order to make use of the DTO DSL, one has to make sure that the OSBP builder is executed after the Xtext builder.
DTO model files
DTO models are described in .dtos
files. These files describe the DTO model and are the basis for the code generation. DTO models may be split over several .dtos
files containing packages in the same namespace.
A .dtos
file may contain several packages with DTOs.
package
Packages are the root element of the DTO DSL grammar. Everything is contained in a
package
; imports, datatypes, DTOs and enums have to be defined within the package. One document can contain multiple packages with unique names.The elements a package can contain are DTOs and enums. Additionally, a package allows
import
statements and the declaration of datatypes.► Syntax:
package name { import importStatement; datatype datatypeDefinition; DTOs }
import
In order to wrap entities into DTOs, the DTO DSL has to reference entities defined in entity model files. Furthermore, it is possible to reference DTOs in other packages. The
import
statement is a way to address these elements without having to use their fully qualified name.Import statements allow the use of the '*' wildcard.
► Syntax:
package name { import importStatement; datatype datatypeDefinition; DTOs }
datatype
The DTO DSL allows the definition of datatypes. These are translated by the inferrer into their standard Java presentation. The behavior of the generator can be controlled by the datatype definitions. There are three types of datatype definitions:
- jvmTypes
- Datatype definitons that map types to jvmTypes take the basic syntax of
datatype <name> jvmType <type> as primitive;
Specifying datatypes in this manner uses an appropriate wrapper class in the generated Java code.
Adding the keywordsas primitive
enforces the use of primitive datatypes where applicable:datatype foo jvmType Integer
compiles to anInteger
whereasdatatype foo jvmType Integer as primitive
results inint
.
- dateTypes
- The datatypes for handling temporal information can be defined by the following statement:
datatype <name> datetype date|time|timestamp;
Datatypes that have been defined in this manner can be used as property variables in DTOs.
- blobs
- Binary blobs can be handled by defining a datatype with the
as blob
keyword. The Java implementation of such a blob is a byte array.datatype <name> as blob;
After
import
statements anddatatype
definitions, the content of the .dtos file is made up of DTO definitions.
DTO
Data Transfer Objects (DTOs) are the most complex elements in the DTO DSL. A DTO wraps an entity defined in the Entity DSL together with its properties and references into a single object used for communication purposes.
DTOs are defined by name, properties, references and wrapper methods. A DTO normally does not implement business logic.
For each DTO that is defined in a package, the DTO DSL automatically generates a Java DTO class and a corresponding mapper class that handles data transfer and conversion between DTO and entity.
► Syntax:
abstract dto <name> extends <parentDto> wraps <entityName> {
dtoFeatures
}
extends
extends
marks a DTO that is derived from another DTO. That means that the properties and references of the parent DTO are inherited.File:Dto-04.pngFigure 4 - Theextends
keyword causes a Java subclass to be created for the DTO.
wraps
wraps
indicates the entity that is handled by the DTO. The entity has to be imported from the entity model file into the DTO package (for importing several entities, the use of the '*' wildcard may be advisable).
Enums
Enums are an abstraction of the Java enum. They compile to enum
classes and can be used as properties in DTOs.
Properties
Properties of DTOs are references to datatypes or enums. They can be regarded as variables and are defined by a keyword followed by a datatype (Java type or datatype defined in the datatype section) and a name.
Properties can be determined with the following keywords:
var
The basic property. Defines a variable that can be used within the DTO. Appropriate getters and setters are created.
id
► -- deprecated -- deprecated -- deprecated -- deprecated -- deprecated -- deprecated -- deprecated -- deprecated --
Defines a field in the DTO for mapping an
id
property (used as a primary key in the persistence layer). This keyword is deprecated. If noid
is given, a warning is shown. Caution: ID autogeneration causes problems, since the value is not set before the entity is persisted to the database, which can cause problems.It is advised to use the
uuid
keyword instead.
uuid
Creates a field for the mapping of Universally Unique IDs as primary keys. UUIDs must be strings.
version
Defines a field for mapping a version property used by the JPA Compiler.
transient
Marks the property as
transient
. Transient properties are not mapped from the DTO to the entity and are thus not persisted. Getter and setter methods are created.
derived
Marks the property as being derived from other values. The logic used in the derivation process may be specified using Xbase. Derived properties are not persisted, and only a getter is created.
References
The DTO DSL tracks relationships between DTOs by using the concept of references. Where appropriate, reverse references can be created using the opposite
property.
References are defined by the ref
keyword, a type and a name. The exact type of reference can be specified as follows:
- multiplicity and nullability: The DTO DSL supports the specification of multiplicities in square brackets appended to the type:
[1]
defines a non-nullable, one-to-one relationship.[0..1]
defines a nullable, one-to-one relationship (this is the default behavior).[1..*]
defines a non-nullable, one-to-many relationship. Anopposite
reference is required.[0..*]
, or simply[*]
, defines a nullable, one-to-many relationship. Anopposite
reference is required.
- opposite reference: In the Entity DSL, lifecycle references between entities need the specification of an
opposite
reference in order to navigate back to the original object after following the reference. The DTO DSL includes a mechanism to map such references using theopposite
keyword. - cascade: The cascade specifier controls the behavior of the database on delete operations. If
cascade
is specified, dependent records are also deleted. In the DTO DSL, these cascading references can be mapped to DTOs by the same keyword.
Inherited Features
Inherited properties and references are the core of the DTO DSL. They provide the mechanism for mapping entities and their features to the respective DTOs and their features.
There are two types of inherited features: inherited properties and inherited references.
Inherited features are defined by the inherit
keyword followed by the feature type and the name of the feature in the entity. After that, the DTO to which a referenced entity or an embedded bean is mapped may be specified using the mapto
keyword.
Finally, custom mapper directives may be specified (see Custom Mappers).
- inherit var: used for mapping entity properties to DTO properties
- inherit ref: used for mapping entity references to DTO references
- mapto: defines the DTO the feature should be mapped to. In the case of inherit var, this keyword is used to point to a DTO that maps a bean from the entity model file. In the case of inherit ref, a DTO must be specified that the reference points to.
► Syntax:
inherit var|ref entityFeature mapto dtoName {customMapper} ;
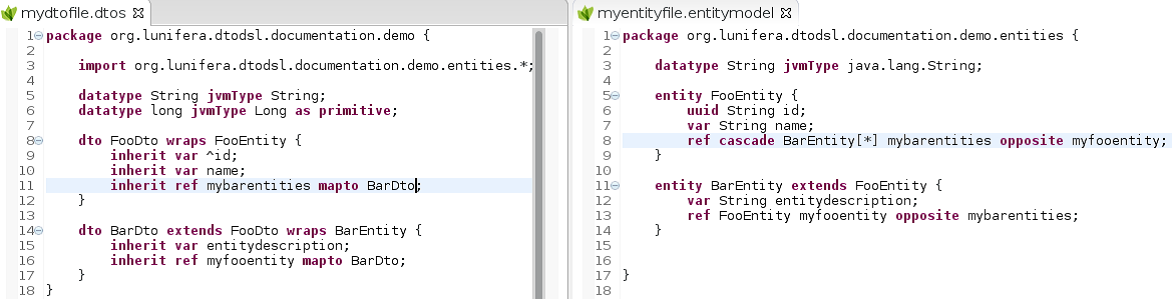
inherit ref
keywords allow for mapping entity references to DTO references. Content assist is available, and multiplicities and opposite references are automatically carried over from the entity model file. The mapto
keyword indicates the DTO that serves as the other end of the reference.Custom Mappers
The DTO DSL provides a mechanism for creating custom mappers between DTOs and entities. These can be defined after the declaration of a property or reference, and they control the information exchange for the respective feature.
In order to create a custom mapper, the toDTO
and fromDTO
keywords are used, followed by the mapper definition in curly braces. toDTO
controls the flow of information from entity to DTO, fromDTO
vice versa. Within the curly braces, the special words in
and out
may be used to reference the source and destination of the mapping.
► Syntax:
inherit var|ref <entityFeature> mapto <dtoName> {
toDto {
XbaseDirectives
}
fromDto {
XbaseDirectives
}
}
Operations
The DTO DSL supports the declaration of operations that can be performed within the DTOs. These operations are based on Xbase and offer a huge set of semantic features, extending the featureset of Java. Xbase additionally offers features like closures. When using operations, bear in mind that it is not good practice to implement business logic within DTOs. Operations can be declared by the def
keyword followed by braces containing the inline operation.
► Syntax:
def returnType operationName() {
XbaseDirectives
}
Comments
Comments can be added anywhere in a .dtos text file and are copied over into the generated Java code. Comments are enclosed in /* ... */
.
Comments before the package
keyword are copied over to all generated Java classes - this is the place for copyright notices.
Copyright Notice
All rights are reserved by Compex Systemhaus GmbH. In particular, duplications, translations, microfilming, saving and processing in electronic systems are protected by copyright. Use of this manual is only authorized with the permission of Compex Systemhaus GmbH. Infringements of the law shall be punished in accordance with civil and penal laws. We have taken utmost care in putting together texts and images. Nevertheless, the possibility of errors cannot be completely ruled out. The Figures and information in this manual are only given as approximations unless expressly indicated as binding. Amendments to the manual due to amendments to the standard software remain reserved. Please note that the latest amendments to the manual can be accessed through our helpdesk at any time. The contractually agreed regulations of the licensing and maintenance of the standard software shall apply with regard to liability for any errors in the documentation. Guarantees, particularly guarantees of quality or durability can only be assumed for the manual insofar as its quality or durability are expressly stipulated as guaranteed. If you would like to make a suggestion, the Compex Team would be very pleased to hear from you.
(c) 2016-2024 Compex Systemhaus GmbH